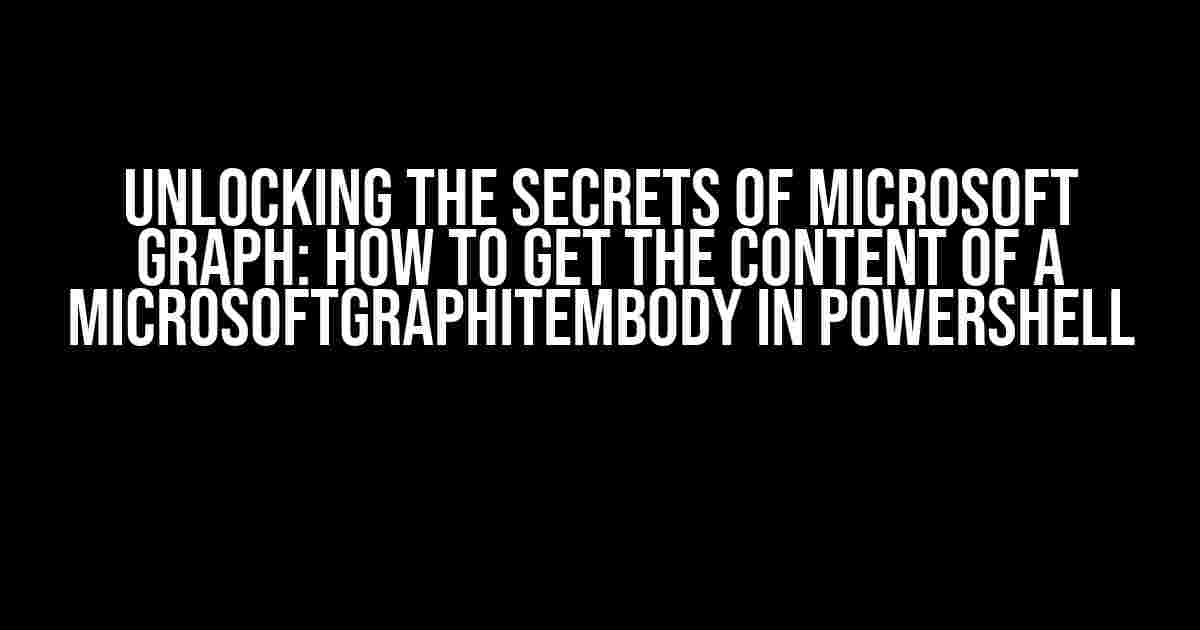
Unlocking the Secrets of Microsoft Graph: How to Get the Content of a MicrosoftGraphItemBody in PowerShell
Posted onAre you tired of navigating the complexities of Microsoft Graph and struggling to extract the content of a MicrosoftGraphItemBody in PowerShell? Fear not, dear reader! In this comprehensive guide, we’ll take you by the hand and walk you through the process of accessing the text content of a MicrosoftGraphItemBody, step by step.
What is Microsoft Graph?
Before we dive into the solution, let’s take a brief detour to understand what Microsoft Graph is. Microsoft Graph is a powerful API that allows developers to access and manipulate data across various Microsoft services, including Office 365, Azure Active Directory, and Microsoft Teams. It provides a unified programmability model that enables developers to build innovative solutions that integrate with Microsoft products.
What is MicrosoftGraphItemBody?
A MicrosoftGraphItemBody represents the body of an item, such as an email message, event, or file, in Microsoft Graph. It contains the content of the item, which can be in various formats, including text, HTML, and markdown. When working with Microsoft Graph in PowerShell, you may encounter scenarios where you need to extract the content of a MicrosoftGraphItemBody. That’s where things can get tricky!
The Challenge: Getting the Content of a MicrosoftGraphItemBody
When you work with Microsoft Graph in PowerShell, you may encounter the MicrosoftGraphItemBody object, which contains the content of an item. However, the content is not directly accessible as a string. Instead, it’s encapsulated within the MicrosoftGraphItemBody object, making it challenging to extract.
The Obstacle: Encapsulation
The primary obstacle is that the MicrosoftGraphItemBody object encapsulates the content, making it difficult to access directly. You can’t simply use the `Select-Object` cmdlet or dot notation to extract the content as a string.
$itemBody = Get-MgItem -ItemId "https://graph.microsoft.com/v1.0/me/mailfolders/inbox/messages"
$itemBody.Content # This will not work!
The Solution: Using the OData-Type
To overcome the encapsulation obstacle, you need to use the OData-type returned by the Microsoft Graph API. Specifically, you’ll work with the `Microsoft.Graph.PowerShell.Models.MicrosoftGraphItemBody` type, which contains the content of the item.
Step 1: Get the MicrosoftGraphItemBody Object
First, you need to get the MicrosoftGraphItemBody object using the `Get-MgItem` cmdlet, which returns an object of type `Microsoft.Graph.PowerShell.Models.MicrosoftGraphItemBody`.
$itemBody = Get-MgItem -ItemId "https://graph.microsoft.com/v1.0/me/mailfolders/inbox/messages"
Step 2: Access the Content Property
Next, you need to access the `Content` property of the MicrosoftGraphItemBody object. This property contains the content of the item as a string.
$itemBody.Content # This will still not work, as the Content property is an OData-type!
Step 3: Use the OData-Type to Extract the Content
To extract the content as a string, you need to use the `Microsoft.Graph.PowerShell.Models.MicrosoftGraphItemBody` type to access the `Content` property. You can do this by casting the `Content` property to a string using the `ToString()` method.
$content = $itemBody.Content.ToString()
Voilà! You now have the content of the MicrosoftGraphItemBody as a string.
Tips and Variations
Here are some additional tips and variations to help you work with Microsoft Graph and PowerShell:
TIP 1: Working with HTML Content
If the content of the item is in HTML format, you may want to extract the plain text content. You can use the `HTML agility pack` NuGet package to parse the HTML content and extract the text.
Install-Package -Name HtmlAgilityPack
$htmlDoc = New-Object HtmlAgilityPack.HtmlDocument
$htmlDoc.LoadHtml($itemBody.Content.ToString())
$textContent = $htmlDoc.DocumentNode.InnerText
TIP 2: Handling Markdown Content
If the content of the item is in markdown format, you may want to convert it to HTML or plain text. You can use the `Markdig` NuGet package to parse the markdown content and convert it to HTML or plain text.
Install-Package -Name Markdig
$markdownParser = New-Object Markdig.MarkdownParser
$htmlContent = $markdownParser Parse($itemBody.Content.ToString())
$textContent = $htmlContent.InnerText
VARIATION 1: Using the `Get-MgItemBody` Cmdlet
Instead of using the `Get-MgItem` cmdlet, you can use the `Get-MgItemBody` cmdlet to retrieve the item body directly. This cmdlet returns the content of the item as a string.
$content = Get-MgItemBody -ItemId "https://graph.microsoft.com/v1.0/me/mailfolders/inbox/messages"
VARIATION 2: Using the `Microsoft.Graph` SDK
Alternatively, you can use the `Microsoft.Graph` SDK to interact with Microsoft Graph in PowerShell. This approach provides more extensive capabilities and flexibility when working with Microsoft Graph.
Install-Package -Name Microsoft.Graph
$graphClient = New-Object Microsoft.Graph.GraphServiceClient
$item = $graphClient.Me.MailFolders.Inbox.Messages.Request().GetAsync().Result
$content = $item.Body.Content.ToString()
Method | Description |
---|---|
`Get-MgItem` | Returns the item object, which contains the `MicrosoftGraphItemBody` object. |
`Get-MgItemBody` | Returns the content of the item as a string. |
`Microsoft.Graph` SDK | Provides extensive capabilities and flexibility when working with Microsoft Graph. |
Conclusion
In this article, we’ve demonstrated how to get the content of a MicrosoftGraphItemBody in PowerShell. By using the OData-type returned by the Microsoft Graph API, you can access the content of the item as a string. We’ve also provided additional tips and variations to help you work with Microsoft Graph and PowerShell.
Remember, when working with Microsoft Graph, it’s essential to understand the structure of the objects and the properties they contain. By following the steps outlined in this article, you’ll be well on your way to unlocking the secrets of Microsoft Graph in PowerShell.
References
- Microsoft Graph API: https://docs.microsoft.com/en-us/graph/use-the-api
- Microsoft.Graph.PowerShell: https://docs.microsoft.com/en-us/powershell/module/microsoft.graph.powershell/
- HTML Agility Pack: https://html-agility-pack.net/
- Markdig: https://github.com/xoofx/markdig
Happy coding, and may the PowerShell be with you!
Frequently Asked Question
Getting the content of a Microsoft.Graph.PowerShell.Models.MicrosoftGraphItemBody in PowerShell can be a bit tricky, but don’t worry, we’ve got you covered!
What is Microsoft.Graph.PowerShell.Models.MicrosoftGraphItemBody, and how does it store content?
Microsoft.Graph.PowerShell.Models.MicrosoftGraphItemBody is a class in the Microsoft Graph PowerShell module that represents the body of an item, such as an email or a Teams message. It stores content as a JSON object, which can be either a plain text or an HTML content.
How can I access the content of a MicrosoftGraphItemBody object in PowerShell?
You can access the content of a MicrosoftGraphItemBody object by using the `Content` property. For example, if you have a variable `$itemBody` that holds an instance of MicrosoftGraphItemBody, you can access its content using `$itemBody.Content`.
Is the content of a MicrosoftGraphItemBody object always a plain text?
No, the content of a MicrosoftGraphItemBody object can be either a plain text or an HTML content. You can check the type of content using the `ContentType` property. If the content type is `Text`, then it’s a plain text, and if it’s `Html`, then it’s an HTML content.
How can I extract the plain text from an HTML content in a MicrosoftGraphItemBody object?
You can use the `ConvertTo-PlainText` cmdlet in PowerShell to extract the plain text from an HTML content in a MicrosoftGraphItemBody object. For example, `$itemBody.Content | ConvertTo-PlainText` will extract the plain text from the HTML content.
Can I modify the content of a MicrosoftGraphItemBody object in PowerShell?
Yes, you can modify the content of a MicrosoftGraphItemBody object in PowerShell. However, keep in mind that modifying the content will also update the underlying item in Microsoft Graph. Make sure to use the `Update-Mg` cmdlet to update the item after modifying its content.